Introduction
C the most popular computer language today was developed from “Basic Combined Programming Languages” (BCPL) called B, in 1972 and implemented at Bell Laboratories . C language possess high interaction with the UNIX operating system.
Program :
A program is set of instructions for performing a particular task. These instructions are just like English words. The computer processes the instructions as 1s and 0s.
Source Program :
A program can be written in assembly language as well as high-level language. This written program is called ‘source program’.
Object Program :
The source program is to be converted to the machine language, which is called ‘Object Program’.
Either an interpreter or compiler will do this activity.
a) Interpreters: An interpreter reads only one line of a source program at a time and converts it to the object codes. In case of errors, the same will be indicated instantly. The program written with an interpreter can easily be read and understood by other users. Therefore security is not provided. Anyone can modify the source code. Hence, it is easier than compilers. However, the disadvantage is that it consumes more time for converting a source program to an object program.
b) Compilers: Compiler reads the entire program and converts it to the object code. It provides errors not of one line but errors of the entire program. Only error-free programs are executed. It consumes little time for converting a source program to an object program. When the program length for any application is large, compilers are preferred.
Structure of a C program :
Every C program contains a number of several building blocks known as functions. Each function performs a task independently. A function is a subroutine that may consist of one or more statements. A C program comprises different sections, which are shown in the following figure.
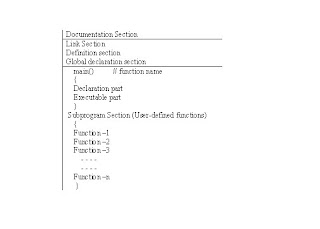
The documentation section consists of the information about the program and author , these information are provided is this section through comment lines. The comment line can be specified in these two different ways.
1. Single line comment – to specify the comment for a single line / *-------*/
2. Multiline comment - to specify the comment for more than one line
/*------------------------------
--------------------------------*/
Example
/* addition program developed by ritch */
main()
{
int a=3,b=8,c;
c=a+b;
printf(“Result= %d”,c);
}
Link Section
Link section provides instructions to the compiler to link functions from the system library.
Example
#include
In this example
Definition Section
The definition section is used to define all symbolic constants.
Example
#define PI 3.14
Global declaration
This section declares some variables that are used in more than one function. These variables are known as global variables. This section must be declared outside of all the functions.
Main() function
Every program written in C language must contain main() function. Empty parenthesis after main is necessary. The function main() is a starting point of every C program. The execution of the program always begins with the function main(). Except the main() function, other sections may not be necessary. The program execution is starts from the opening brace ({) and ends with the closing brace (}). Between these two braces, the programmer should declare declaration and executable parts.
Declaration part: The declaration part declares the entire variables that are used in the executable part. Initializations of variables are also done in this section. Initialization means providing initial value to the variables.
Executable part: This part contains the statements following the declaration of the variables. This part contains a set of statements or a single statement. These statements are enclosed between braces.
Sub program Section The functions defined by the user are called user-defined functions. These functions are generally defined after the main() function. They can also be defined before main() function. This portion is not compulsory.
Importance of C :
Ø C is a robust language with rich set of built-in functions.
Ø Programs written in C are efficient and fast.
Ø Very close to machine and user.
Ø It provide variety of data type and powerful operator.
Ø C is highly portable.
Ø C language is well suited for structured programming.
Ø It is much faster than BASIC.
Ø Several standard functions are available.
Ø Ability to extend itself.
Programming Rules:
1. All statements should be written in lowercase letters. Uppercase letters are only used for symbolic constants.
2. Blank spaces may be inserted between the words. This improves the readability of the statements. However, this is not used while declaring a variable, keyword, constant and functions.
3. It is not necessary to fix the position of a statement in the program, that is, the programmer can write the statement anywhere between the two braces following the declaration part. The user can also write one or more statements in one line separating them with a semicolon(;). Hence, it is often called a free-form language.
The following statements are valid.
a=b+c;
d=b*c;
or
a=b+c;d=b*c;
4. The opening and closing braces should be balanced, that is, for example, if opening braces are four then closing braces should be four.
Executing the program :
The following steps are essential in executing a program in C.
a) Creation of a program: The program should be written in C editor. The file name does not necessarily include the extension ‘.C’. The default extension is ‘.C’. The user can also specify his/her own extension.
b) Compilation and linking a program: ( To compile: Alt + F9 ) The source program statements should be translated into object program, which is suitable for execution by the computer. The translation is done after correcting each statement. If there is no error, compilation proceeds and the translated program is stored in another file with the same file name with extension ‘.obj’. If at all errors are there the programmer should correct them. Linking is also an essential process. It puts all other program files and functions which are required by the program together. For example, if the programmers is using pow() function, then the object code of this function should be brought from math.h library of the system and linked to the main program.
c) Executing the program: ( To execute: Ctrl+F9 ) After the compilation, the executable code will be loaded in the computer’s main memory and the program is executed.
The C Character set :
The characters are used to form words, numbers and expressions depending upon the computer on which the program runs. The characters in C are classified into the following categories.
1) Letters 2) Digits 3) White spaces 4) Special characters
Character Set:
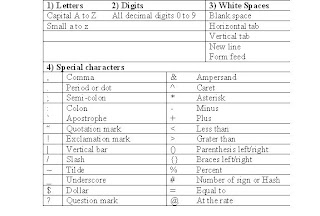
Language patterns of C uses special kinds of symbols, which are called as delimiters. They are
Keywords or Reserved words :
All the C keywords have been assigned fixed meaning. The keywords cannot be used as variable names because they have been assigned fixed jobs.
C Tokens :
The smallest individual unit such as identifier in a program is called token .
The token can be any one of these as given below
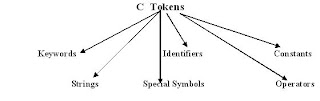
Identifiers are names given to variables, functions, and arrays and other user-defined objects .
Rules to form an Identifiers:
The identifiers are formed with letters and digits and special characters , (_) underscore.
The first character must be an alphabet.
No special characters are allowed except underscore.
Upper and lower case are distinct.
Keywords are not allowed.
Valid Identifiers
A0, Basicpay, Total_T
InValid Identifiers
AB. – Special character (.) not allowed
9A - The first character must be an alphabet.
Break - Keywords are not allowed.
Constants :
The constants in C are applicable to the values, that do not change during the execution of the program. C constants are declared by using const keyword. There are several types of constants in C.
Example : const int a=20; is value 20 to a cannot be modified.
1) Integer constants: These are the sequence of numbers from 0 to 9 without decimal points or fractional part or other symbols. It requires minimum two bytes and maximum four bytes.
Example
10, +20, -15
2) Real Constants: Real constants are often known as floating point constants. Integer constants are unfit to represent many quantities. For example, length, height, prize, distance etc. are measured in real numbers.
Example
2.5, 5.521, 3.14 etc.
1.4561 * e+3 ( exponential notations are allowed )
B) Character Constants :
1) Single character constants: A character constant is a single character. They are also represented with a single digit or a special symbol or white space enclosed within a pair of single quote marks.
Example
‘a’, ‘8’, ‘ ‘ , etc.
Character constants have integer values known as ASCII values.
Example
printf(“%c %d”, 65, ‘B’) will display the characters ‘A’ and 66.
2) String constants: String constants are a sequence of characters enclosed within a double quote marks. The string may be a combination of all kinds of symbols.
Example
“Hello”, “India”, “444”, “a”
Backslash Character Constants or Escape sequence :
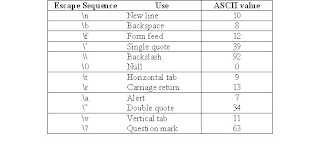
Variables :
A variable is a data name used for storing a data value. Its value may be changed during the program execution. The variable’s value keeps on changing during the execution of a program. In other words, a variable can be assigned different values at different times during the execution of a program.
Example
height, average, sum, etc.
Rules for defining variables:
1) They must begin with a character without spaces but an underscore is permitted.
2) The length of the variable varies from compiler to compiler. Generally most of the compilers support 8 characters excluding extension. However, the ANSI Standard recognizes the maximum length of a variable upto 31 characters.
3) The variable should not be a C keyword.
4) The variable names may be a combination of upper and lower characters. For example, sum or suM are not the same.
5) The variable name should not start with a digit.
Declaring Variables :
The declaration variables should be done in the declaration part of a program. The variables must be declared before they are used.
Syntax
Data_type variable_name
Example
int age;
char m;
float a,b,c;
Initializing Variables :
Variables declared can be assigned or initialized using an assignment operator ‘=’. The declaration and initialization can also be done in the same line.
Syntax
variable_name= constant
or
Data_type variable_name= constant
Example
x=2;
int y=2;
int x=y=z=2;
‘C‘ Data Types :
C language is rich set in its data types. Storage representation and machine instructions to handle constants differ from machine to machine. The variety of data type appropriate to the needs of the application as well as the machine . ANSI C supports four classes of data types.
1) Basic data type (or) primary data type
2) Derived type
3) User-defined type
4) Empty data set
1. Primary data types : All C compilers support four fundamental data types. Namely,
1. char -character in the character set -1 byte
2. int -an integer -2 byte
3. float -a single-precision floating point number -4 byte
4. double -a double-precision floating point number -4 byte
Data type modifiers or Qualifiers
Data types type modifiers are used to modify the basic data types. And to produce new data types,
signed
unsigned
long
short
These can be used with integer and character data types only. Long is used with double.
Integer Types
Integers are whole numbers with a range of values supported by a particular machine. Generally integers occupy one word of storage, and since the word of machines vary(16 or 32 bits) the size of an integer that can be stored depends on the computer. The size of the integer value is limited to the range -32768 to 32767. C has three classes of integer storage, namely short int, int, long int, in both signed and unsigned forms.
Example
int a,b,c;
Floating point types
Floating point (or real) numbers are stored in 32 bits, with 6 digits of precision. Floating point numbers are defined in C by the keyword float .
Example
float a,b,c;
Double types
When the accuracy provided by a float number is not sufficient , the type double can be used to define the number. These are known as double. To extend the precision further, we may use long double which uses 80 bits.
Example
double a,b,c;
Character types
The single character can be defined as a character(char) type data. Characters are usually stored in 8 bits( one bytes) of internal storage. The qualifier signed or unsigned may be explicitly applied to char.
Size and Range of Data types on a 16- bit machine
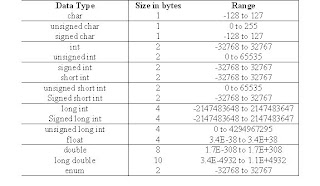
C allows users to define and identifier to represent an existing data type
Syntax
typedef int a;
a b,c,d;
now in the line b,c,d are declared as an integer data type.
Enumerated data type
The other user defined data type is enumerated data type
Syntax
enum identifier{value1,Value2………Valuen.}
Example
enum day{mon,tue,wed,thu,fri,sat,sun}
enum day week;
The variable week can have the value any one from the list mon – sun.
3. Derived Data Type:
The derived data types specifies the pointers, functions , arrays, structures. Where we are collecting variables of different type and deriving it to a new type.
a) Pointers: A pointer is a memory variable that stores a memory address. Pointers can have any name that is legal for other variables and it is declared in the same fashion like other variables but it is always denoted by ‘*’ operator.
Example
int *x; float *f; char *y;
b) Functions: A function is a self-contained block or a sub-program of one or more statements that perform a special task when called.
c) Arrays: An array is a collection of similar data types in which each element is located in separate memory location.
Example
int b[4];
4.Empty data set
The empty data set describes about function i.e user defined or main function.
Type Conversion
The lower type is automatically converted to the higher type.
int i,x;
float f;
double d;
long int l;
If any operand is of type char then it would be converted to int.
All floats are converted to double.
If either operand is double, then the other is converted to a double and the result produced is also a double.
If one of the operands is unsigned long int, then the other will be converted to unsigned long int and the result will be unsigned long int.
5. If one of the operands is long int and the other is unsigned int then, if possible
unsigned int will be converted to long int to produce a long int. If this is not
possible, then both operands will be converted to unsigned long int to produce a
unsigned long int result.
Operators and Expressions :
C supports a rich set of operators . An operators is a symbol that tells the computer to perform certain mathematical or logical manipulations. Operators are used in programs to manipulate data variables. C operators can be classified into eight categories,
Arithmetic Operators
Relational Operators
Logical Operators
Assignment Operators
Increment and Decrement Operators
Conditional Operators
Bitwise Operators
Special Operators
Size of Operator
1.Arithmetic Operators :
Arithmetic operators are used to do arithmetic processing. These operators and their meanings are listed below.
The Operator which is used for numerical calculations between the two values.
a) Arithmetic Operators
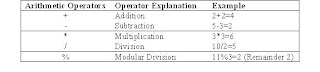
Integer arithmetic:
In an arithmetic expression if both the operands are integer operands then that expression is in as an integer expression. And the operation is called integer arithmetic,
Example a=14, b=4
a + b = 18 a – b = 10 a * b = 56
a / b = 3 (decimal part truncated) a % b = 2 (remainder of division)
Real arithmetic:
In an arithmetic expression if both the operands are real operands then that expression is an real arithmetic expression.
Example
x = 6.0/7.0 = 0.857143
y = 1.0/3.0 = 0.333333
Mixed-mode arithmetic:
When one of the operand is real and the other is integer. The expression is called mixed-mode arithmetic expression. Operand is real type, then only the real operation is performed and the result is always real number. Thus
15/10.0 = 1.5
15/10 = 1
Program /* Arithmetic operator without using variables */
#include
main( )
{
int a,b;
clrscr():
printf(“Enter two numbers\n”);
scanf(“%d%d”,&a,&b);
printf(“A+B=%d\n”,a+b);
printf(“A-B=%d\n”, a-b);
printf(“AxB=%d\n”, a*b);
printf(“A/B=%d\n”,a/b);
getch();
}
output:
Enter two numbers
20,10
A+B=30,
A-B=10,
A*B=300,
A/B=2
2.Relational Operators:
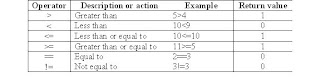
#include
main( )
{
int a,b;
clrscr( );
printf(“Enter two numbers\n”);
scanf(“%d%d”,&a,&b);
if(a>b)
printf(“A is Big\n”);
else
printf(“ B is Big\n”);
getch( );
}
output:
Enter two numbers
25,15
A is Big
3.Logical Operators:

Note:
1) The Logical AND (&&) operator provides true result when both expressions are true otherwise 0.
2) The Logical OR () operator provides true result when one of the expressions is true otherwise 0.
3) The Logical NOT (!) operator provides 0 if the condition is true otherwise 1..
Program /* Logical operators finding out biggest among 3 numbers */
#include
#include
main()
{
int a,b,c;
clrscr();
printf(“\n Enter Three numbers \n”);
scanf(“%d%d%d”,&a,&b,&c);
if((a>b)&&(a>c))
printf(“A is Big\n”);
if((b>a)&&(b>c))
printf(“B is Big\n”);
if((c>b)&&(c>b))
printf(“Cis Big\n”);
getch();
}
output:
Enter three numbers
25,15,20
A is Big
4.Assignment Operators:
Assignment operator are used to assign the result of an expression to a variable. Usual assignment operator is ‘=’ . C has a set of ‘shorthand’ assignment
Syntax:
vop=exp
Where v is a variable, exp is an expression , op is a C binary arithmetic operator.
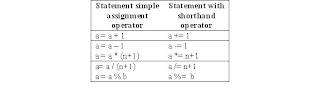
Program /* program for assignment operators */
#include
main()
{
int a,b;
a=10,b=15;
printf(“%d%d\n”,a,b);
}
output:
10
15
Program /* program for show use of shorthand operators */
#include
main()
{
int a,b;
a=10,b=15;
a+=1;
b+=1;
printf(“%d%d\n”,a,b);
}
output:
11
16
5.Increment and Decrement Operators:
C has two very useful operator not generally in other languages. These are the increment and decrement operator.
+ + and --
the operator + + adds 1 to the operand while - - subtract 1. both are unary operator and the following form
++m or m++
- -m or m- -
++m; is equivalent to m = m+1; ( or m+=1;)
--m; is equivalent to m = m-1; ( or m-=1;)
Program /* increment and Decrement operator*/
#include
main()
{
int a=10,b,c,d;
b=++a;
c=a++;
d=--a;
printf(“ A is =%d\n”,a);
printf(“ B is =%d\n”,b);
printf(“ C is =%d\n”,c);
printf(“ D is =%d\n”,d);
getch();
}
output:
A is = 11
B is = 11
C is = 11
6.Conditional Operators / Ternary Operator :
A ternary operator pair “ ? : “ is available in C to construct conditional expression of the form
Syntax :
exp1? exp2:exp3;
where exp1,exp2,exp3 are expression.
The operator ?: work as follows exp1 is evaluated first. If it is nonzero (true), then the expression exp2 is evaluated. If exp1 is false, exp3 is evaluated.
Consider the following statements.
a=10;
b=15;
x=(a>b)?a:b;
in this example, x will be assigned the value of b.
Program /* Ternary operator */
#include
main()
{
int a=10,b=15,c;
c=(a>b)?a:b;
printf(“\n c value is %d”,c);
getch();
}
output:
c value is 15
7.Bitwise Operators :
Bitwise operator are used for testing the bits. Or shifting them right or left. Bitwise operator may not be applied to float or double.
These operators can operate only on an integer operands such as int, char, short, long int etc.
Program /*Example program to shift inputted data by two bits right*/
#include
#include
main()
{
int x,z;
clrscr();
printf(“\n Read the integer from keyboard (x):”);
scanf(“%d”,&x);
x>>=2;
y=x;
printf(“The right shifted data is =%d”,y);
}
output:
Read the integer from keyboard (x) : 8
The right shifted data is =2
Shifting two bits right means the inputted number is to be divided by 2s where s is number of shifts. (ie) in short y=n/2s
Where, n=number
S=number of position to be shifted
As per the program as cited above
Y=8/22 =2
Program /*Example program to shift inputted data by three bits left*/
#include
#include
main()
{
int x,z;
clrscr();
printf(“\n Read the integer from keyboard (x):”);
scanf(“%d”,&x);
x<<=3; y=x; printf(“The left shifted data is =%d”,y); } OUTPUT: Read the integer from keyboard (x) : 2 The left shifted data is =16 The corresponding decimal number is 16 i.e. answer should be 16. Shifting three bits left means number is multiplied by 8 in short y=n*2s Where, n=number s=number of position to be shifted As per the program as cited above Y=2*22 =16 Table of AND: INPUTS OUTPUT X Y Z 0 0 0 0 1 0 1 0 0 1 1 1 Program /*Example program to use bitwise AND operator between the two integers and display the results.*/ #include
#include
main()
{
int a,b,c;
clrscr();
printf(“\n Read the integers from keyboard (a & b):”);
scanf(“%d %d”,&a,&b);
c=a&b;
printf(“The answer after ANDing is =%d”,c);
}
output:
Read the integers from keyboard (a & b) : 8 4
The answer after ANDing is (C)=0
Program /*Example program to use bitwise OR operator between the two integers and */display the result.
#include
#include
main()
{
int a,b,c;
printf(“\n Read the integers from keyboard (a & b):”);
scanf(“%d %d”,&a,&b);
c=ab;
printf(“The ORing operation between a & b in c =%d”,c);
getch();
}
output:
Read the integers from keyboard (a & b) : 8 4
The ORing operation between a & b in c=12
Program /*Example program with Exclusive OR operator between the two integers and display the result*/
#include
#include
main()
{
int a,b,c;
clrscr();
printf(“\n Read the integers from keyboard (a & b):”);
scanf(“%d %d”,&a,&b);
c=a^b;
printf(“The data after Exclusive OR operation is in c =%d”,c);
getch();
}
output:
Read the integers from keyboard (a & b) : 8 2
The data after Exclusive OR operation is in c=10
Table of Inverter
INPUT (X) OUTPUT (X)
0- 1
1- 0
8.Special Operator :
C supports some special operator of interest such as comma operator, pointer operator(& and *) and member selection operator(. And ->).
i)Comma Operator:
Comma operator(,) : The Comma Operator is used to separate two or more expressions.
Example : a=2, b=5 , c=a+b;
v=(x=10,y=5,x+y);
first assign the value 10 to x, then assign value 5 to y, finally assign 15.(ie.10+5) to v. since comma operator has the lowest precedence of all operator.
Program /*Example program to comma operator*/
#include
main()
{
int a,b,c;
clrscr();
printf(“\n Enter Two number : \n”);
scanf(“%d%d”,&a,&b);
c=(a=15,b=15,a+b);
printf(“\n result is =%d”,c);
}
output:
result is = 30
9.The Sizeof Operator:
The sizeof operator is a compile time operator and, when used with an operand, it return the number of bytes the operand occupies. The operand may be a variable, a constants or a data type qualifier.
Example:
m=sizeof(sum);
n=sixeof(long int);
k=sizeof(2345);
Program /*Example program of sizeof operator*/
#include
main()
{
int a,b,c;
clrscr();
a=sizeof(sum);
b=sixeof(long int);
c=sizeof(12345);
printf(“\n byte is =%d\n%d\n%d\n”,a,b,c);
}
output:
byte is =2
byte is =4
byte is =3
Arithmetic Expressions :
An arithmetic expression is a combination of variables and constants, and operator arranged as per syntax of language.
Evaluation of Expressions:
Expressions are evaluated using an assignment of the form
Syntax
Variable=expression;
No comments:
Post a Comment